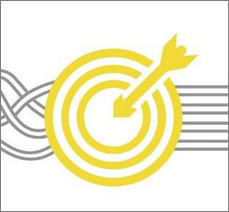
I saw this on AngelikaLanger.com and wanted to extract the following portions for my own reference/reminder:
From the java.io.File
source code, we have the following:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
| public File[] listFiles(FileFilter filter) {
String ss[] = list();
if (ss == null) {
return null;
}
ArrayList<File> files = new ArrayList<>();
for (String s : ss) {
File f = new File(s, this);
if ((filter == null) || filter.accept(f)) {
files.add(f);
}
}
return files.toArray(new File[files.size()]);
}
|
The above method takes FileFilter
as a parameter. The FileFilter
interface is:
1
2
3
| public interface FileFilter {
boolean accept(File pathname);
}
|
An example usage of this interface (and the listFiles()
method) is:
1
2
3
4
5
6
7
8
9
10
| File myDir = new File("/user/admin/deploy");
if (myDir.isDirectory()) {
File[] files = myDir.listFiles(
new FileFilter() {
public boolean accept(File f) {
return f.isFile();
}
}
);
}
|
The above example uses an anonymous class. We can re-work the above example to use a lambda expression instead of an anonymous class:
1
2
3
4
5
6
| File myDir = new File("/user/admin/deploy");
if (myDir.isDirectory()) {
File[] files = myDir.listFiles(
(File f) -> { return f.isFile(); }
);
}
|
We can do this, in this specific case, because FileFilter
is a functional interface (it only has one method to be implemented).
We can go further (in this specific example) and use a method reference instead of the lambda expression:
1
2
3
4
| File myDir = new File("/user/admin/deploy");
if (myDir.isDirectory()) {
File[] files = myDir.listFiles( File::isFile );
}
|