A simple, self-contained WebSockets
demo where messages generated by Javalin
are sent to a web page containing a DataTable
. It’s so basic, it’s not even bi-directional.
The complete code is on GitHub here
.
The demo uses stock symbols (e.g. AAPL
) as unique identifiers within JSON messages, and randomly generated prices - for example:
JavaScript
1
2
3
4
|
{
"symbol": "AAPL",
"price": 67
}
|
Each message is added to the table (if it does not already exist), or is used to update the price in the table:
JavaScript
1
2
3
4
5
6
7
8
9
10
11
12
13
|
function updateTable(message) {
let stockData = JSON.parse(message.data);
// check if symbol already in table:
var index = table.column( 0, {order:'index'} ).data()
.indexOf( stockData.symbol );
if (index >= 0) {
// update the existing row:
table.row( index, {order:'index'} ).data( stockData ).draw();
} else {
// insert a new row:
table.row.add( stockData ).draw();
}
}
|
The page is displayed at http://localhost:7070/ticker
.
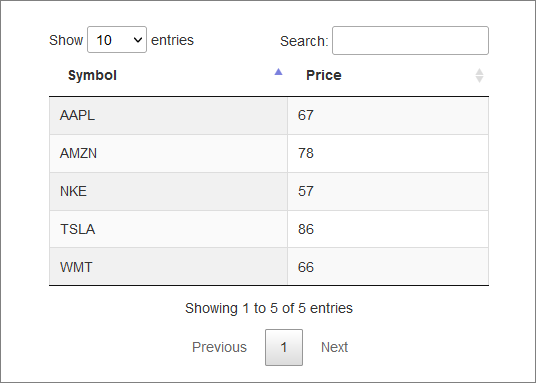
![]()
The websocket itself is opened at ws://localhost:7070/tickerfeed
.
Java:
Java
1
2
3
4
5
|
app.ws("/tickerfeed", ws -> {
ws.onConnect(ctx -> { ... });
ws.onMessage(ctx -> { ... });
ws.onClose(ctx -> { ... });
});
|
JavaScript:
JavaScript
1
2
|
let ws = new WebSocket("ws://" + location.hostname + ":" + location.port
+ "/tickerfeed");
|